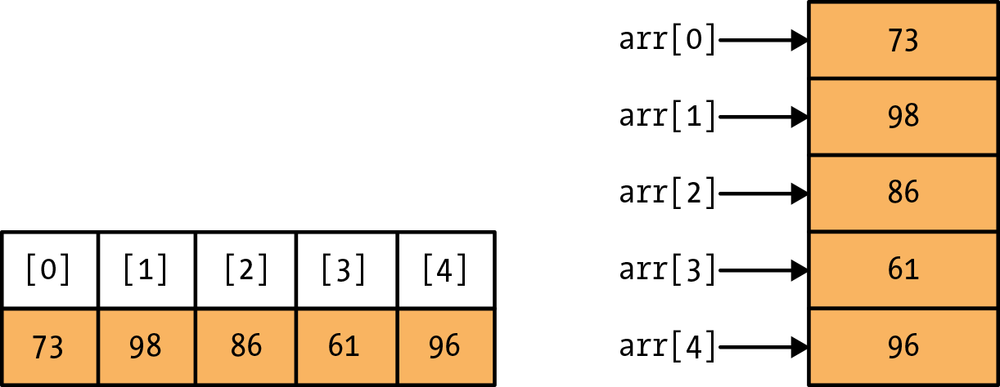
What is an Array?
Arrays are a popular type of data structure.
What is a Data Structure?
In computer science, a data structure is a data organization, management and storage format that enables efficient access and modification.[1][2][3] More precisely, a data structure is a collection of data values, the relationships among them, and the functions or operations that can be applied to the data.
Wikipedia
In the simplest terms: data structure stores data in an organized way!
How Arrays Store Data
In the array data structure, data values are stored at specific locations. Each location is identified by a number (index). Location or indices (plural of index) start at 0.
Example:
- Location 0 stores the text “Jason”
- Location 1 stores the text “Roberta”
- Location 2 stores the text “Jessie”
….
Simple right?
Naming Arrays
But how do we reference this array data structure? In other words what do we call this specific array?
Let’s give it a name. Looking at the values it looks like we are storing names so call it ‘names‘? I think that makes sense.
names = [0 –> “Jason” , 1 –> “Roberta“, 2 –> “Jessie”]
So ‘names‘ refers to [Jason, Roberta and Jessie].
How it Looks in Code
Let’s pick javascript as the programming language for the programming examples. This is how the ‘names’ array looks in javascript.
var names = ["Jason", "Roberta", "Jessie"];
Still with me? Pretty straight forward right? I bet you can tell me what is stored in ‘names’ array at location 1. If you said “Roberta” you are correct! If you said “Jason” you must have forgotten that locations start at 0 not 1 so location 0 is “Jason”.
Accessing Values in an Array
What if you wanted to access the value at location 2? This would be the same as the third item in the ‘names’ array since location start at 0 not 1. 0 is the first value, 1 is the second value, 2 is the third value.
We want the value of names at location 2
names[2];
names[2] returns the value “Jessie”
Changing Values in an Array
What if you wanted to change the value in an array? Simple.
names[0] = "Abdul";
Now names looks like [Abdul, Roberta, Jessie]
Adding a Value to an Array
names[3] = "Alita";
Location 2 stored the last item in the array. So we pick location 3 to store the new item. Nothing was stored in location 3 so we should be good.
names looks like [Abdul, Roberta, Jessie, Alita] now
Removing a Value in an Array
Don’t ask. It’s not as straightforward as adding or modifying. All you need to know that there are built in function that can be used to accomplish the task. If you just can’t let go try googling ‘javascript splice function to remove element‘.
More on Arrays
Arrays are a popular data structure. They are simple and efficient. We used text or string for the value type. You can use other types such as numbers or ints. And if you use arrays in javascript you can mix the types. Also, in most programming languages arrays have built-in functions that return various things such as the length of an array or the last item in an array.
Conclusion
Arrays are a popular data structure. They are efficient and well organized. They make working with a data set a breeze. They become even more appealing once you understand and work with its built-in functionalities.
Feel free to comment your concerns below or reach out to me if you are interested in learning more about arrays or any other data structure for that matter!
Happy Coding!
Leave a Reply