Here is how you can quickly add a member to your Mailchimp mailing list through PHP and using the Mailchimp API.
Step 1 – GET API Helper File
Go to https://github.com/drewm/mailchimp-api
Grab the MailChimp.php inside the src folder
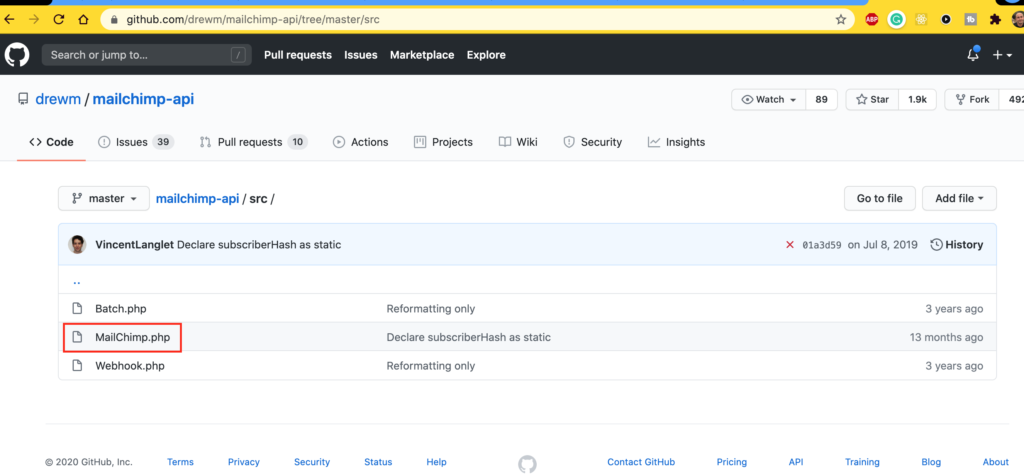
Place it in the same directory where you run your PHP code that handles your form submissions (if you have such an action).
Step 2 – Hide Your MailChimp API Key + List ID
create a keys.php file somewhere outside of the public folder. In my case I had my website in /home/freeirvl/public_html so I placed the keys.php in /home/freeirvl
Your keys.php should look something like
<?php
$mailchimp_api_key = 'YOUR KEY HERE';
$mailchimp_list_id = 'YOUR LIST ID HERE';
?>
Step 3 – The Actual PHP Code!
In whatever file you are trying to add a member to your MailChimp mailing list do the following:
// Load credentials file
require_once '/home/freeirvl/keys.php';
Load the MailChimp helper/wrapper file
// ************************************ LOAD MAILCHIMP LIBRARY************************************
include(dirname(__FILE__) . '/MailChimp.php');
use \DrewM\MailChimp\MailChimp;
// ************************************ END LOAD MAILCHIMP ************************************
The function that will actually try add a member using the helper/wrapper file. Feel free to change the argument list. In the following example I decided to take in an email address ($email), first name ($first_name) and last name ($last_name). You might want to leave out first name and last name.
Also don’t forget to actually CALL on this function somewhere in your code and provide it with the correct values!
// ************************************ MAILCHIMP ************************************
function subscribe_add_tags($email, $first_name, $last_name) {
// *************** LOADING API KEY AND LIST ID
global $mailchimp_api_key, $mailchimp_list_id;
$MailChimp = new MailChimp($mailchimp_api_key);
$list_id = $mailchimp_list_id; // <--- PLAYGROUND LIST_ID
$hashed= md5(strtolower($email));
// *************** CHANGE TAG NAME HERE
$payload = '{ "tags": [ { "name": "Bootcamp", "status": "active" } ] }';
$payload_json = json_decode($payload, true);
$result_subscribe = $MailChimp->post("lists/$list_id/members", [
'email_address' => $email,
'status' => 'subscribed',
'merge_fields' => array(
'FNAME' => $first_name,
'LNAME' => $last_name,
)
]);
$result_tag = $MailChimp->post("lists/$list_id/members/$hashed/tags", $payload_json);
// this error success produces NOTHING in the Response from MailChimp
// because it doesn't seem to send anything, but if not error then success I guess
// so you can still capture its status
if ($MailChimp->success()) {
print_r($result_subscribe);
print_r($result_tag);
} else {
echo 'Error Subscribing and or Tagging Mailchimp<br>';
print_r($result_subscribe);
print_r($result_tag);
echo $MailChimp->getLastError();
}
} // end function
// ************************************ END MAILCHIMP ************************************
That is it.
Notes: I am adding a tag to this member! Also, google how to obtain the MailChimp mailing list id if you are confused about what that is exactly. I have also created a video version of this tutorial.
Let me know your thoughts or if I forgot to mention anything.
For tutoring feel free to visit my tutoring site https://avantutor.com or post your questions on https://askavan.com
Leave a Reply