So you downloaded an amazing website template that you just can’t wait to work with. You were able to customize the whole template except for the contact form part. You realize that it takes some backend work in order for it to function. The problem is you are not a backend person. You just need some clear instructions on how to get the contact form data to be emailed to yourself. Well, here it is – assuming you have hosting with cPanel and an email associated with your domain through your host provider.
The HTML Form
<form method="post" action="php/contact_us_action.php">
<div class="form-group row">
<div class="col-md-6 mb-4">
<input type="text" class="form-control" placeholder="First name" name="first_name">
</div>
<div class="col-md-6">
<input type="text" class="form-control" placeholder="Last name" name="last_name">
</div>
</div>
<div class="form-group row">
<div class="col-md-12">
<input type="text" class="form-control" placeholder="Subject" name="subject">
</div>
</div>
<div class="form-group row">
<div class="col-md-12">
<input type="email" class="form-control" placeholder="Email" name="email">
</div>
</div>
<div class="form-group row">
<div class="col-md-12">
<textarea class="form-control" id="" cols="30" rows="10" placeholder="Write your message here." name="message"></textarea>
</div>
</div>
<div class="form-group row">
<div class="col-md-6">
<input type="submit" class="btn btn-primary py-3 px-5 btn-block" value="Send Message">
</div>
</div>
</form>
What is important here is the name attribute for each form element. Your form will probably look different and have different fields but you definitely need to add a name attribute to each form element. Additionally, pay special attention to the following line:
<form method="post" action="php/contact_us_action.php">
action attribute specifies where to handle the email submission logic. In this case I created a ‘php’ folder and then created a contact_us_action.php file. This is where the main form submission takes place.
The contact_us_action.php File
Once the user submits the form we go to contact_us_action.php. Please create this file (inside the newly created php folder) and add the following code to it.
<?php
// [ATTENTION] Change this if you want to check for a different field
// Program doesn't continue if email field is missing
if(isset($_POST['email'])) {
// EDIT THE 2 LINES BELOW AS REQUIRED
$email_to = "CHANGE_TO_YOUR_PERSONAL_EMAIL_ADDRESS;
function died($error) {
// your error code can go here
echo "We are very sorry, but there were error(s) found with the form you submitted. ";
echo "These errors appear below.<br /><br />";
echo $error."<br /><br />";
echo "Please go back and fix these errors.<br /><br />";
die();
}
// validation expected data exists
// [ATTENTION] This part makes sure that 'first_name', 'message' and
// 'email' fields were submitted. Add more or remove some.
if(!isset($_POST['first_name']) ||
!isset($_POST['message']) ||
!isset($_POST['email'])) {
died('We are sorry, but there appears to be a problem with the form you submitted.');
}
// [ATTENTION] Change these fields accordingly.
// Change the value for $email_from_default
$first_name = $_POST['first_name'];
$last_name = $_POST['last_name'];
$subject = $_POST['subject'];
$message = $_POST['message'];
$email_from = $_POST['email'];
$email_subject = "CHANGE_TO_YOUR_SUBJECT";
$email_from_default = "CHANGE_TO_YOUR_HOST_DOMAIN_EMAIL";
$error_message = "";
$email_exp = '/^[A-Za-z0-9._%-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,4}$/';
if(!preg_match($email_exp,$email_from)) {
$error_message .= 'The Email Address you entered does not appear to be valid.<br />';
}
$string_exp = "/^[A-Za-z .'-]+$/";
if(!preg_match($string_exp,$first_name)) {
$error_message .= 'The First Name you entered does not appear to be valid.<br />';
}
if(!preg_match($string_exp,$last_name)) {
$error_message .= 'The Last Name you entered does not appear to be valid.<br />';
}
if(strlen($error_message) > 0) {
died($error_message);
}
$email_message = "Form details below.\n\n";
function clean_string($string) {
$bad = array("content-type","bcc:","to:","cc:","href");
return str_replace($bad,"",$string);
}
$email_message .= "First Name: ".clean_string($first_name)."\n";
$email_message .= "Last Name: ".clean_string($last_name)."\n";
$email_message .= "message: ".clean_string($message)."\n";
$email_message .= "Email: ".clean_string($email_from)."\n";
// create email headers
$headers = 'From: '.$email_from_default."\r\n".
'Reply-To: '.$email_from_default."\r\n" .
'X-Mailer: PHP/' . phpversion();
@mail($email_to, $email_subject, $email_message, $headers);
?>
<!DOCTYPE html>
<html lang="en">
<head>
<link rel="shortcut icon" href="images/icon.ico">
<title>CHANGE_TO_YOUR_TITLE</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<meta name="description" content="Thank you for contacting us" />
</head>
<body>
<h1>Thank You!</h1>
</body>
</html>
<?php
}
?>
In the example above the form has a first_name, last_name, subject, email and a message field. Change it to whatever fields you have. I have added [ATTENTION] comment to all the places where you have to pay special attention to and might have to do an edit. Also pay attention to all CHANGE_… text. Feel free to modify the HTML section at the end.
PHP mail Function and Your Host
I am using the PHP mail function to achieve my goal. There are other ways! I believe they require more work but none the less there are other ways. In order for the mail function to work out of the box I needed to make sure I had an email account that was created in the Namecheap cPanel and that was hosted on their servers.
Here is how determined what my default email address with my host was. Login into your cPanel then click on Email Accounts
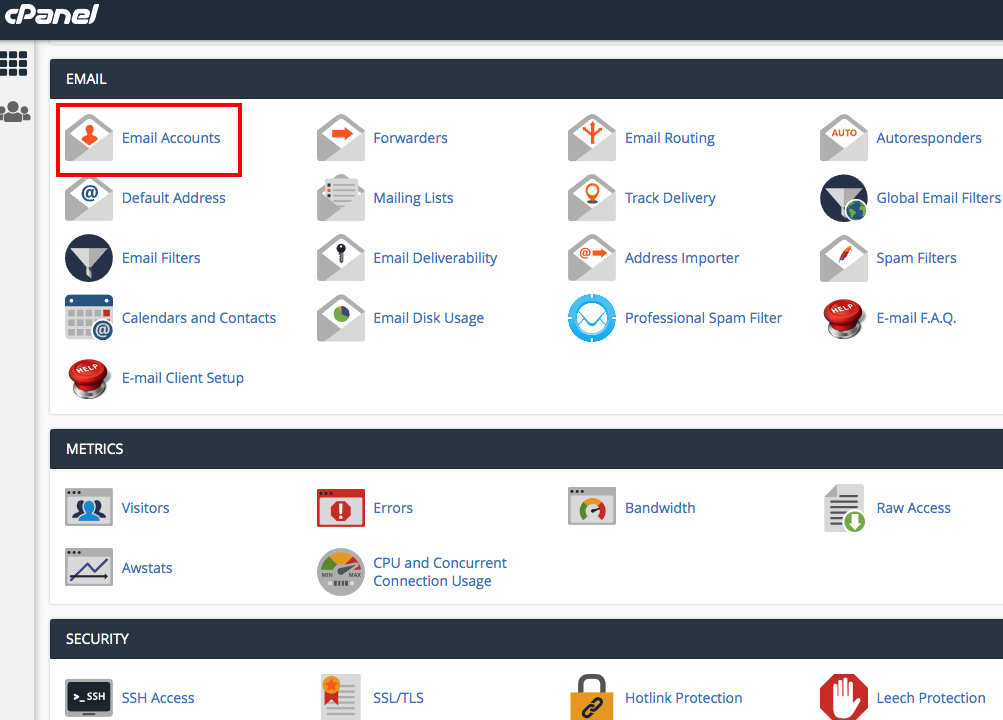
I had an email account already listed. If you don’t have one just click on create otherwise click on CHECK EMAIL
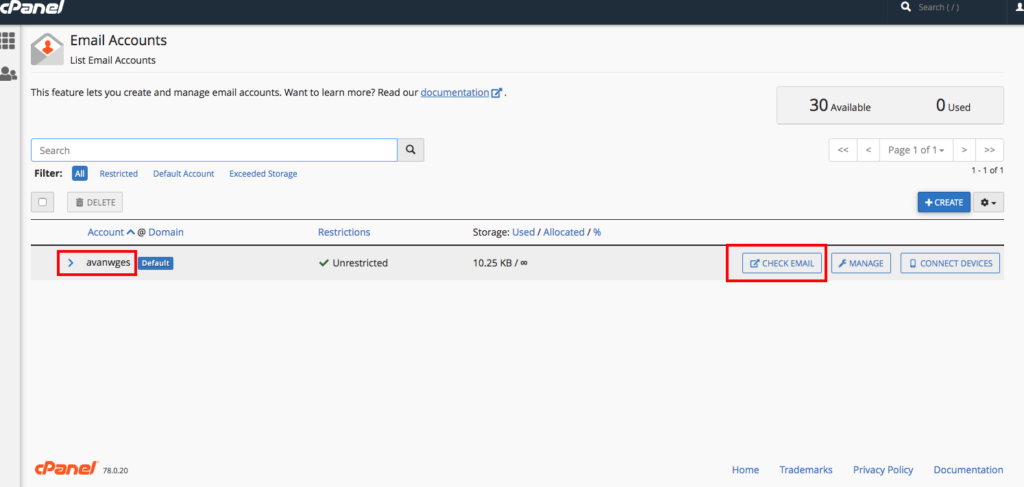
Click on horde
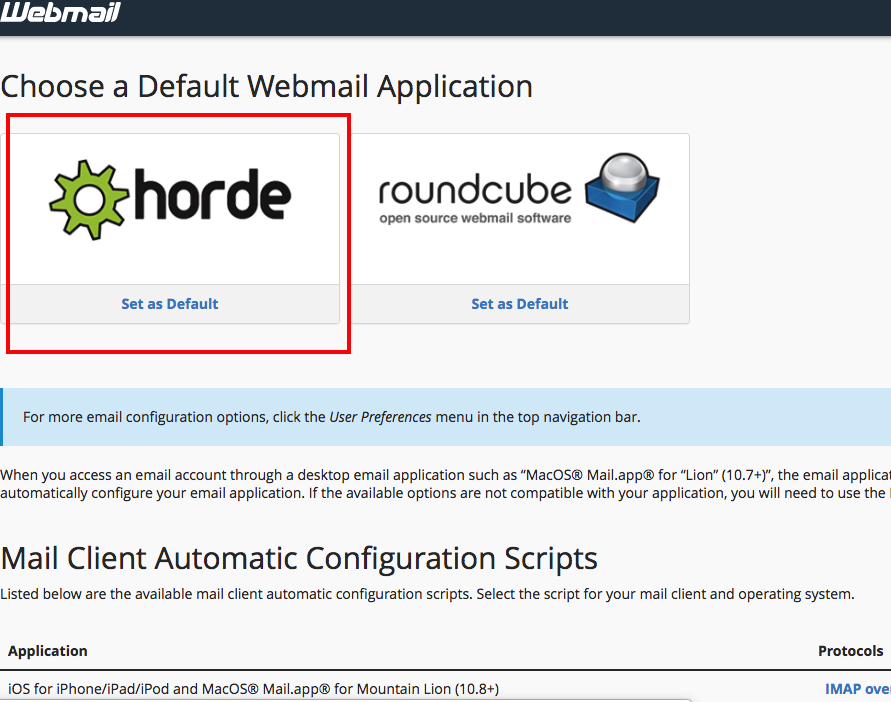
Send an email to personal email address here
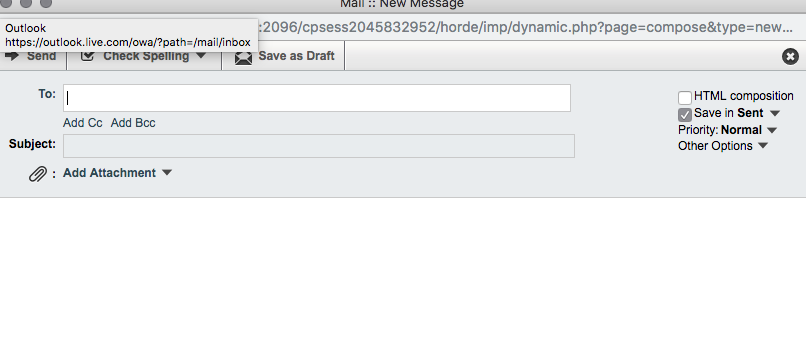
Check your personal email inbox and copy the from email address. That is one way to obtain what the full email address associated with your host is. I am sure there is an easier way but this is how I did it. In my case it wasn’t as simple as username@mydomain.com.
My host Namecheap also suggested I have Local Mail Exchanger (whatever that is). Here is how I made sure I do! Click on Email Routing in cPanel.
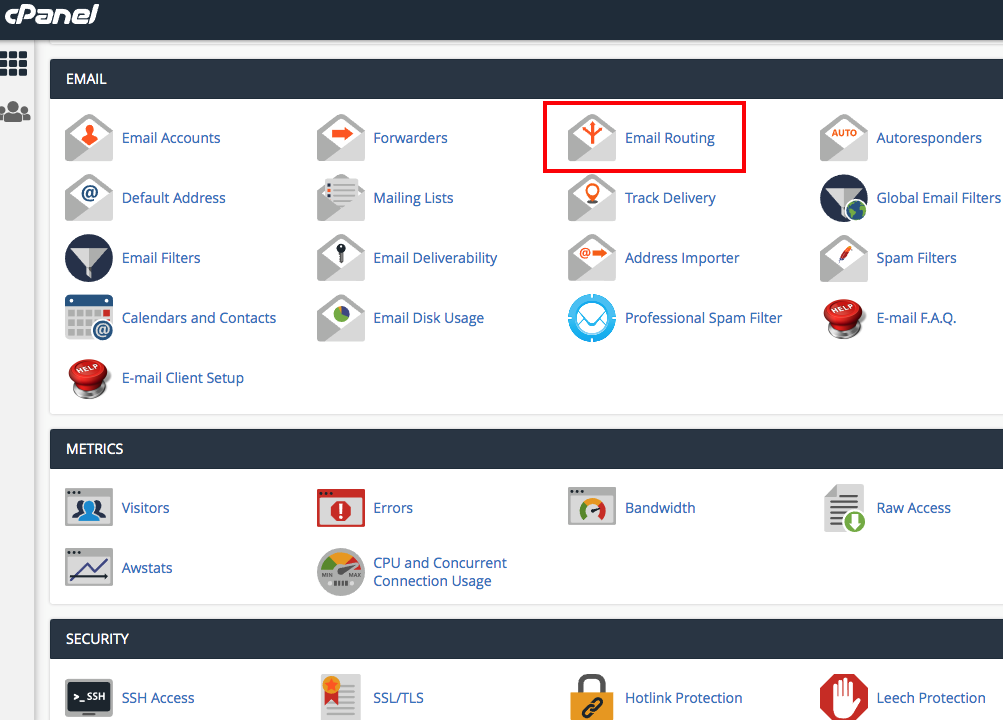
Bamn, that it is. It was already selected for me.
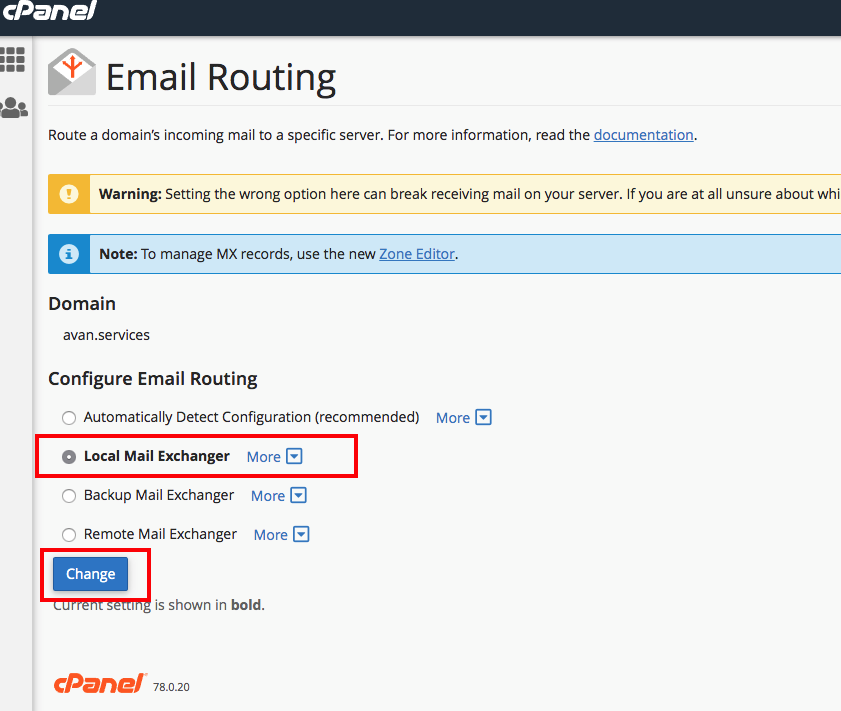
Upload to Host and Done
Once I had uploaded my modified files including the new contact_us_action.php file onto the server I was able to use the contact us form. I didn’t have to install special PHP email libraries and mess with 3rd party email configurations which was awesome.
If you are looking for more information I recommend checking out the following namecheap article:
Good Luck and Happy Coding!
Leave a Reply