Here what I did to add a ‘I am not a robot’ reCAPTCHA box to my PHP form.
Step 1 (Register Site with reCAPTCHA)
Go to https://www.google.com/recaptcha/admin
Register your site
Select reCAPTCHA v2Verify requests with a challenge
Then select “I’m not a robot” Checkbox
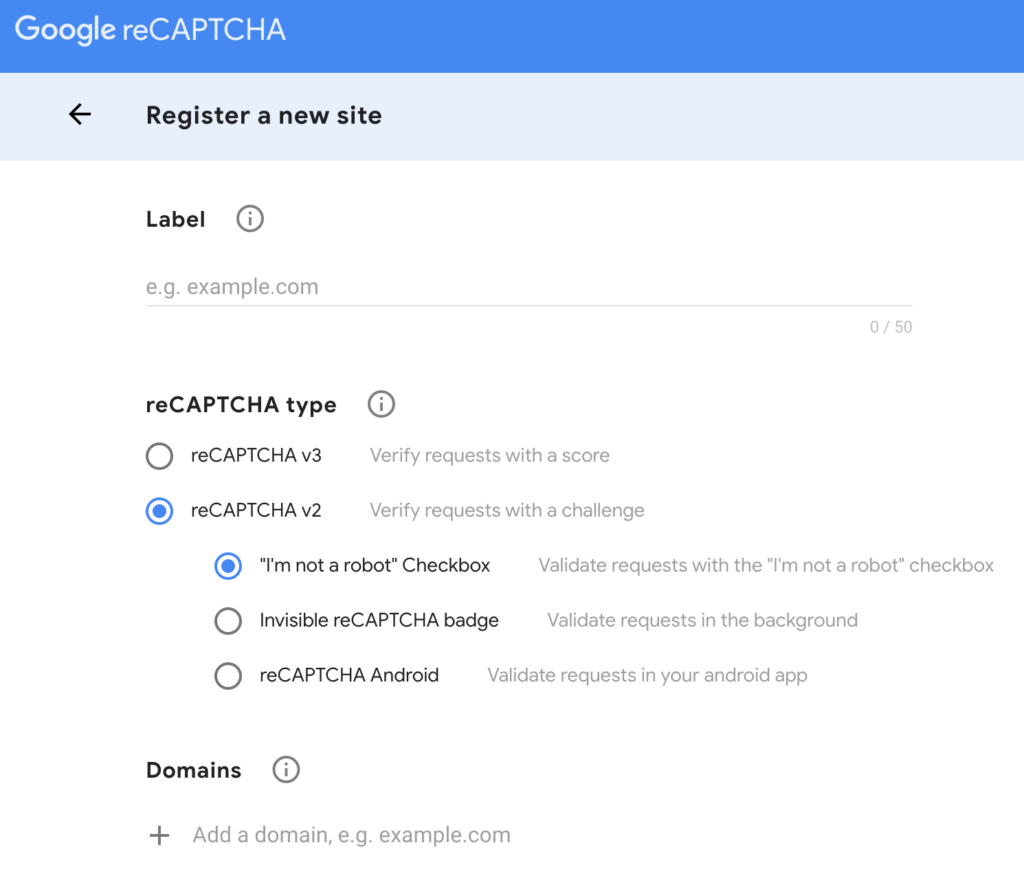
Step 2 (Get Site Key and Secret Key)
Take note if your SITE and SECRET KEYS
Go to settings in your reCAPTCHA admin console and click on reCAPTCHA keys
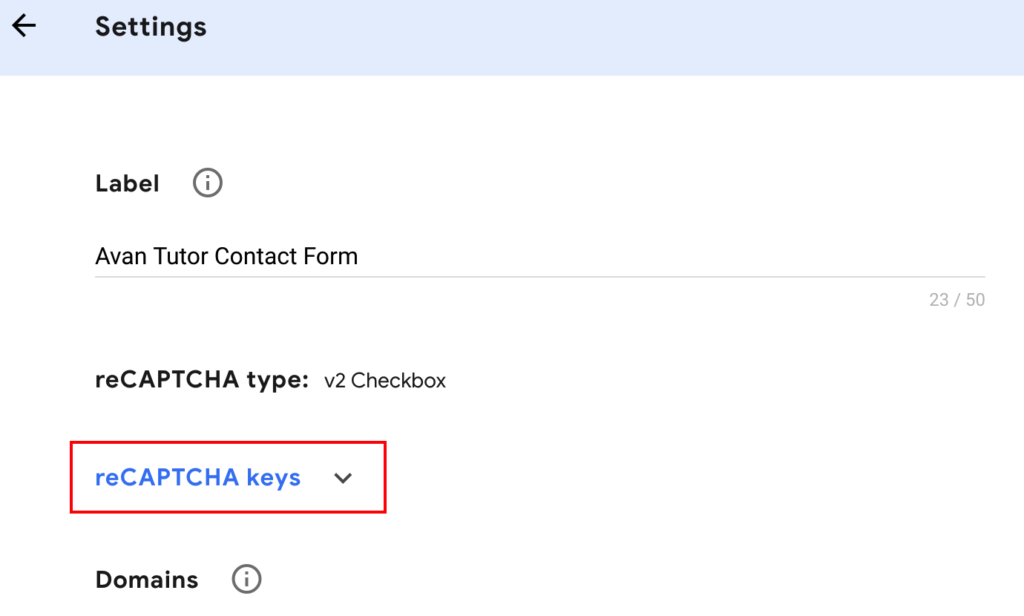
Keep the following page open or temporarily copy keys to a text editor
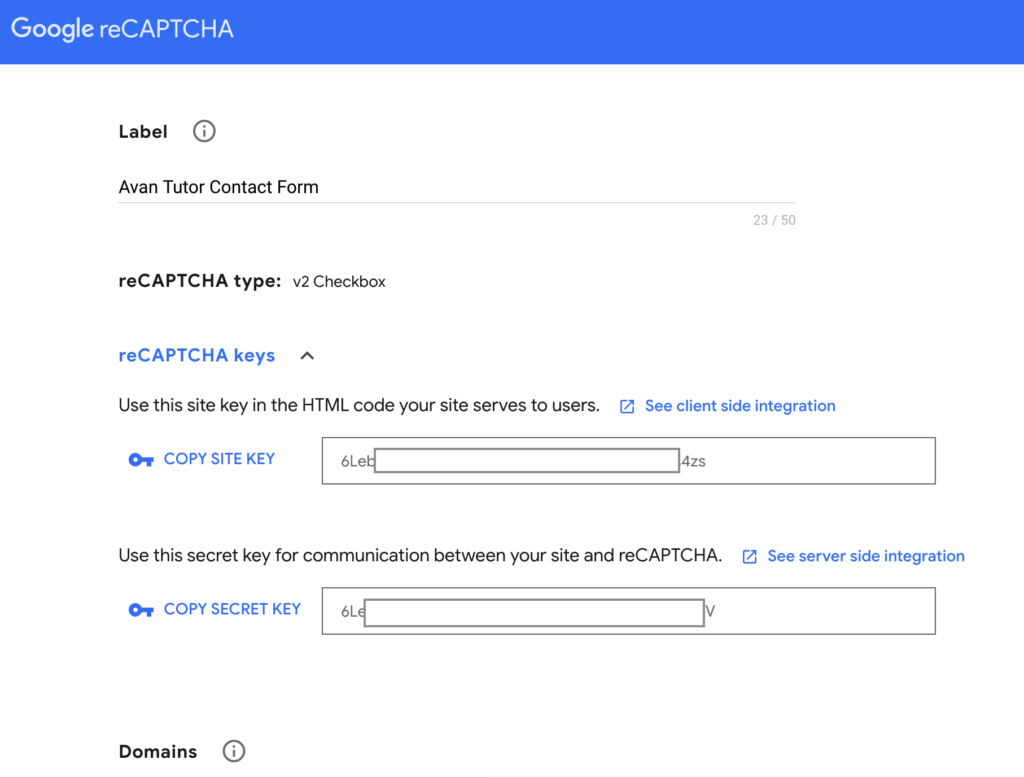
Step 3 (Add Script to Front End)
Add reCAPTCHA javascript inside your head tags
<head>
...
<script src="https://www.google.com/recaptcha/api.js"></script>
...
</head>
Add reCAPTCHA box inside your form tag. Don’t forget to INSERT YOUR SITE KEY
<form name="FORM_NAME" method="post" action="DESTINATION_ACTION">
...
<div class="g-recaptcha" data-sitekey="INSERT_YOUR_SITE_KEY"></div>
...
</form>
Step 4 (BACKEND PHP)
In your DESTINATION_ACTION php file insert the following code block. Don’t forge to INSERT YOUR SECRET KEY. Not your site key!
if(isset($_POST['g-recaptcha-response'])) {
// RECAPTCHA SETTINGS
$captcha = $_POST['g-recaptcha-response'];
$ip = $_SERVER['REMOTE_ADDR'];
$key = 'INSERT SECRET KEY HERE';
$url = 'https://www.google.com/recaptcha/api/siteverify';
// RECAPTCH RESPONSE
$recaptcha_response = file_get_contents($url.'?secret='.$key.'&response='.$captcha.'&remoteip='.$ip);
$data = json_decode($recaptcha_response);
if(isset($data->success) && $data->success === true) {
}
else {
die('Your account has been logged as a spammer, you cannot continue!');
}
}
When human validation fails the user sees the following message
“Your account has been logged as a spammer, you cannot continue!”
Bam What!
Leave a Reply